Introduction to Node.Js and React.js
What is React.js?
React.js is a JavaScript library used for creating dynamic and interactive web user interfaces. It was developed by Facebook and is used for building modern web applications. React.js is built around a concept called "components", which are reusable and independently managed pieces of code that make web application development easier and more efficient.
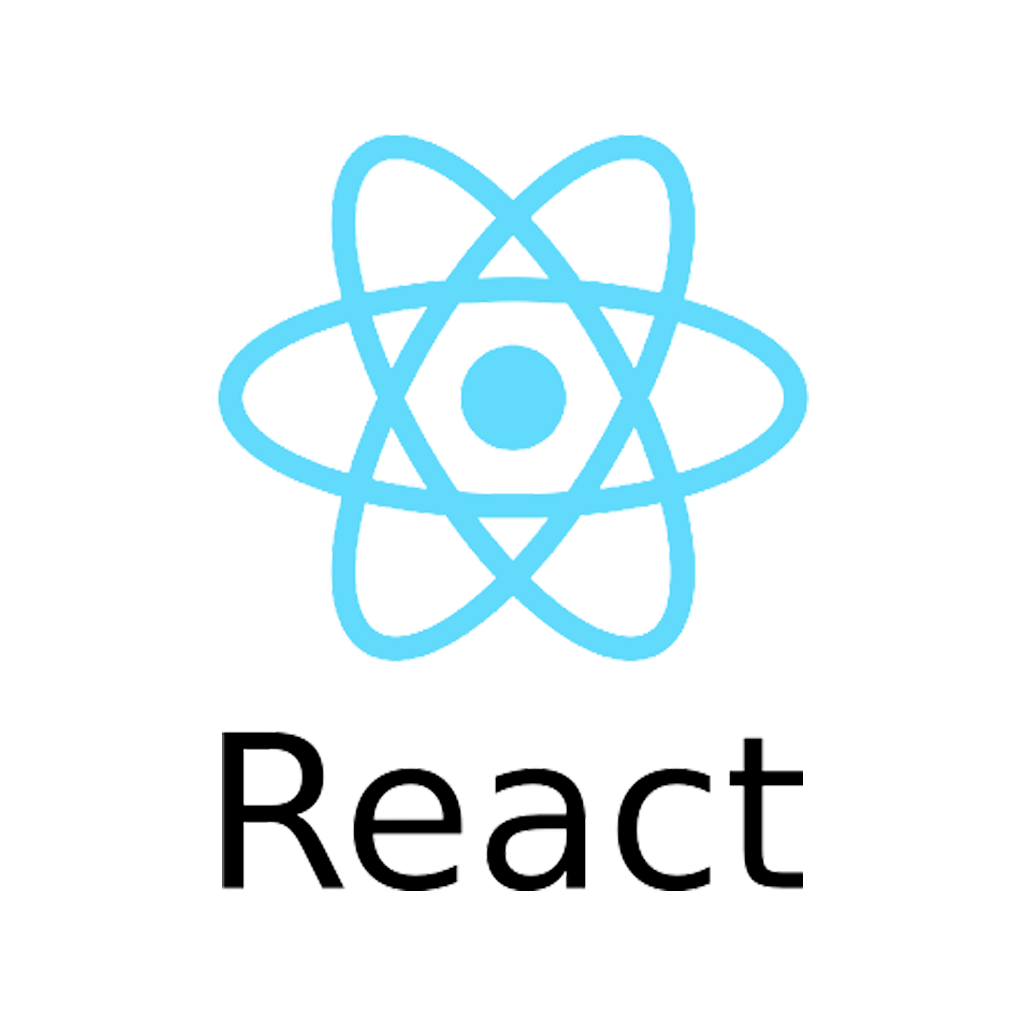
Why use React.js?
There are several reasons to use React.js in web application development. Here are some of the most important:
- Code reuse: React.js encourages developers to create reusable components, which can significantly reduce development time and lead to cleaner and more maintainable code.
- Performance: React.js has excellent performance due to the fact that it uses Virtual DOM, a technique that optimizes the way web pages are updated without requiring a full page reload.
- Large community: React.js has a large and active community of developers who share knowledge and develop additional tools and libraries that can be used with React.js.
What makes React.js different from other frameworks?
React.js differs from other frameworks by its modular and component-based approach. While other web frameworks focus on building entire web pages, React.js focuses on building reusable components. This modular approach makes development easier and more efficient because developers can build and test components independently, then use them in different web applications without having to rewrite them. React.js also uses the Virtual DOM to handle page updates, making apps faster and more efficient than those built using other frameworks.
Node.Js
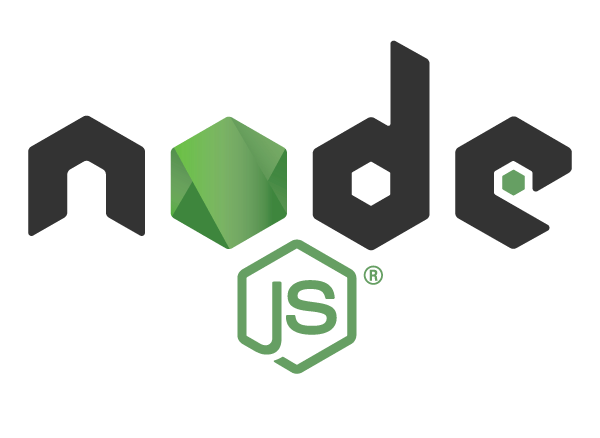
To work with React.js you first need to install Node.Js and create a project. Node.Js is a JavaScript/TypeScript runtime originally thought to be used for running JavaScript code on the server with the idea that web developers can work with only one language and for small to medium projects or for prototyping it is a suitable development environment for a product. Node.Js is built with the same Javascript engine, V8, that Chromium-based browsers also use.
However, Node.Js is also used for SPA (Single-Page Application) frontend web applications. Here Node.Js is used to compile frontend applications with the help of libraries or frameworks such as React.js, Angular or Vue.js that will be packaged in a minified JavaScript file (the size of the script file is minimized) to be served by to a server. The application will be requested by a browser, the application file will be loaded and the browser will dynamically render the web page based on the JavaScript code. It should be noted that Node.Js for these applications is only used for development and compilation.
Node.Js comes bundled with a couple of utilities, npx and npm. These are used to work with the various JavaScript/TypeScript libraries that you can also find on npmjs.
We will first use the npx utility to create the first React project, this utility is used to run various commands from NPM (Node Package Manager) packages as follows:
npx create-react-app my-app --template typescript
There are other project templates with different build tools for React projects with different advantages such as:
npm create vite@latest my-app -- --template react-ts # for Vite
npx create-next-app@latest # for Next.js
This command will create a minimal React project already initialized with a package.json file and a few components. This JSON file is a configuration file for the project that declares the NPM packages used and the commands scripts to run for Node.Js. To install new packages you can directly issue the following command in the directory with this JSON file:
npm install jwt-decode
Or add the package to package.json with the desired version in the `dependencies' field and giving the command:
npm install
The installation command will initialize or update the package-lock.json file, it is required to exist. It is used so that people working on the same project work with exactly the same version of libraries so that they can be installed instead of the exact versions of compatible version packages and there are no discrepancies between development environments when working on the project.
In package.json there are also scripts to be run by Node.Js in the scripts
field:
{
"scripts":
{
"start": "node ./index.js"
}
}
This startup script can be invoked like this:
npm run start
To help you with the project you have here a basic project to start from to implement your frontend for the project.
React components
You'll notice that in a React app visual components are declared in JSX/TSX (JavaScript/TypeScript XML) files. Basically, in these files the script is interspersed with HTML components (it is improperly said but they can be treated as normal HTML tags). This helps developers define their visual components as easily as in normal HTML code but by declaring component logic more easily directly in script.
In React, components are fundamental building blocks of the user interface. They are reusable and can be combined to create complex interfaces. There are two types of components in React:
- Class-based components: These are components that are written as classes and extend the React.Component class. These components have access to properties and state of the component, as well as various React lifecycle methods.
class HelloWorld extends React.Component {
render() {
return <h1>Hello, World!</h1>;
}
}
- Functional components: These are components that are written as functions and return a TSX component that describes how the component should look.
function HelloWorld() {
return <h1>Hello, World!</h1>;
}
const HelloWorld = () => {
return <h1>Hello, World!</h1>;
}
We recommend using functional components instead of the class-based version because special functions called "hooks" are used a lot and for this reason it tends towards a functional approach for React code.
Properties (props) and state (states) in React.js
Properties (props) are arguments you can pass to components. These are used to customize the behavior of a component and can be accessed via the props object.
Example of using properties in a functional component:
function Welcome(props) {
return <h1>Hello, {props.name}!</h1>;
}
const element = <Welcome name="John" />;
ReactDOM.render(element, document.getElementById('root'));
Here, name
is a property passed to the Welcome
component. This property can be accessed in the component via the props object
State is another important feature of React components. State is used to manage component data that may change over time. The state is defined by the hook function useState which returns for an initial state the object with the state and a function to change the state.
Example of using state in a class-based component:
import React, { useState } from 'react';
const Counter = () => {
const [state, setState] = useState({ count: 0 })
return <div>
<p>Count: {state.count}</p>
<button onClick={() => setState({ count: state.count + 1 })}>
Increment
</button>
</div>
}
ReactDOM.render(<Counter />, document.getElementById('root'));
303 / 5,000 In this example, the state is defined by useState and represents the number of button presses. The button triggers the setState method, which updates the state and causes the component to re-render. Any change to the state object directly does not trigger its harsh re-rendering of the component.
What are hook functions?
Hook functions are special functions that are called one after another in the same order when a component is re-rendered. With the help of these functions, it is very easy to implement complex logic for the UI independent of the visual component by composing such more complex functions from simpler hook functions. These functions must be called with use
in front just like useState
and can receive data like any normal function and return data that can come from calling other hook functions.
Hook functions must be called in the same order each time and cannot be called conditionally, for example, called inside an `if' block, but can contain conditionally called blocks of code.