HTML and CSS in the context of React
HTML
HTML (HyperText Markup Language) is the standard markup language used in web applications. In order to develop web applications, developers need to know HTML. An HTML file is composed like any XML file from a syntax tree in the form of nested standard HTML elements. An HTML element is formed:
- From a start tag "<tag_name>"
- A trailing tag "</name_tag>"
- Attributes specified in the opening tag <tag_name attribute_name1="attribute_value1" attribute_name2="attribute_value2">
- Child elements specified between start and end tag, elements without children can be specified <tag_name />
An HTML file has the following general structure:
<!DOCTYPE html> <!-- Document type declaration -->
<html> <!-- HTML Code Declaration -->
<head> <!-- Metadata, scripts, styles for elements and some elements to be displayed in the browser are declared in the head element -->
<title>Page Title</title> <!-- The title element declares the title of the page to be displayed in the tab in the browser -->
</head>
<body> <!-- In the body we declare the elements to be displayed in the page rendered by the browser -->
<h1>My First Heading</h1> <!-- We can declare a text heading with h1-6 tags -->
<p>My first paragraph.</p> <!-- We can declare paragraphs with the p --> element
<a href="https://en.wikipedia.org/wiki/HTML">My first link</a> <!-- Here we have a link text that takes as attribute the URL to which reference is made -->
</body>
</html>
Since HTML is a very broad language we will refer to this tutorial for more details. However, to work with various web libraries or frameworks it is only necessary to know the general structure of an HTML element. In React, for example, components are used exactly as HTML elements with attributes and child elements, developers can define their own components under this form using predefined components that are then translated into classic HTML elements. Thus, developers only have to define in modular the component with the application component. Additionally, there are various libraries like Materia UI that expose already customized and styled components.
However, there are slight differences in the way things work in a TSX file in React compared to the regular HTML code declaration:
import React, { PropsWithChildren } from 'react';
import ReactDOM from 'react-dom/client';
const MyLink = (props: PropsWithChildren<{ text: string, url: string }>) => {
return <div>
<a href={props.url}>{props.text}</a>
{props.children}
</div>
}
ReactDOM.render(<MyLink text='This is a link and a text below' url='https://google.com'>
<p>Text as a child</p>
</MyLink>, document.getElementById('root'));
Notice that the values of attributes and elements that are inserted into components are declared using with the variables declared in the function. Also, the attributes are passed to the component exactly like the attributes and children of the component are referenced by the children field given by the PropsWithChildren type which also adds this field to the type given as a genericity parameter.
CSS
Using HTML we can declare how the application will look visually, but we want to customize the appearance of the web application. For this reason, to overcome the limitations of the HTML language, the CSS (Cascading Style Sheets) standard was created to style components such as what color to use, how to align or what text font to use. The CSS structure is as follows:
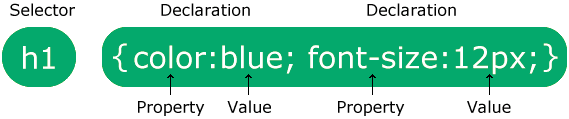
The declaration is a key-value dictionary where you specify which property is styled with which value. The statement is preceded by a selector, it can be:
- the universal selector * that applies to all elements
- the list of elements over which the style is applied, e.g.
p,div,h1
- the id of an element where
id
is given as an attribute, e.g.#test
is applied to all elements with theid="test"
attribute - the class of an element where
class
is given as an attribute, e.g..test
applies to all elements with theclass="test"
attribute - the class of an element type, e.g.
p.test
applies to allp
elements with theid="test"
attribute - we can also use selectors for the descendants of a component with
>
, e.g.div > .center
will apply the style to the descendants of adiv
element that has thecenter
class
There are other types of special selectors, but we won't go into details here.
p {
text-align: center;
color: red;
}
#para1 {
text-align: center;
color: red;
}
.center {
text-align: center;
color: red;
}
p.center {
text-align: center;
color: red;
}
div > .center {
text-align: center;
color: red;
}
Declared styles can also be inserted inline in HTML code but are generally recommended to be included in separate CSS files.
<p style="font-size: 100px;">Large font<p>
Of course, the CSS standard is quite vast, and we recommend going through the following tutorial for more details. The most difficult thing about working with CSS for a developer is figuring out which properties to apply to different elements in the application, the properties being very diverse.
In React, many libraries come with already styled components, but these can also be customized. For that, you can directly import the .css file into the TSX component to apply the style like for example:
import "./HomePage.css"
Unlike HTML, in a TSX file it should be noted that the word class is reserved in JavaScript/TypeScript and for this reason the attribute to declare the class of an element is className instead of *class *.
You can also declare inline styles but with the note that they become objects in the TSX file:
<p style={{fontSize: "100px"}}>Large font<p>
SASS and SCSS
For more advanced styling you can use SASS (Syntactically Awesome Stylesheet), this is a CSS proprocessor that has some advantages over CSS normal like being able to declare variables and import variables. With the help of SASS, the styling of the application can be modularized more easily than in the case of simple CSS. To use SASS the NPM package must be installed:
npm install sass
SASS files with the .sass extension can be imported exactly like CSS files into the component where they are used. Older SASS syntax looks like this:
@import ./otherColors
$colorRed: #FF0000
p.center
text-align: center
color: $colorRed
Or they can be declared closer to CSS syntax as SCSS (Sassy CSS) files with the extension .scss:
@import "./otherColors";
$colorRed: #FF0000;
p.center {
text-align: center;
color: $colorRed;
}
Tailwind
There are alternatives to CSS like Tailwind. Tailwind is a framework over the CSS that facilitates the definition of styles directly in HTML without the need to write separate CSS files.
Tailwind has the advantage of being easier to understand and easier to apply than CSS explicitly and facilitates the implementation of a responsive UI when resizing the screen. The disadvantage is that it is explicitly applied to each component where it is needed. To use Tailwind you only need to apply to the className
attribute (for React, for HTML in general is the class
attribute) the names of the classes corresponding to the necessary styles.
The previous example can be implemented with Tailwind in react as follows:
<p className="text-center text-red-500">This is a paragraph<p>